Lesson 1: Using the command line & creating our first c++ file
In the previous lesson we learnt what the main function was, and what it looks like in code. In this lesson we are going to create a new file for our code, write our main function in there, and compile it. A source code file in C++ has the extension .cpp, just a text file has the extension .txt or a song has the extension .mp3. There's nothing special about a .cpp file, it's just text, it can't even store fonts or font size, it just stores the text characters. So to create one we can open up our trusty command line. If you don't remember how to do this go to Lesson 2 in Setup.
Once it's open we're going to create a folder where we want to write our programs. To do this we're going to learn some new command line commands.
1. Firstly decide where you want to store your programs, this might be in Documents or on the Desktop. Where ever you choose we need to know the root address of it i.e. where it exists on the computer. For example my Documents folder exists at C:\Users\olive\Documents. So its on the C: drive (then the back slash means go to the next folder), then in the Users folder, go into olive folder, then in that folder you'll find Documents.
On Mac it's a slightly different setup, your documents will be at ~/Documents. This special swiggly character (tilde character) means your user folder. Other folders like Desktop, Applications, Downloads will also be in here.
2. The next step is using the cd command. This is without a doubt the most useful command to know. It's an abbreviation of change directory. Basically the command line is always at some directory. For example when you start it up, it most likely will start in your User directory. You can always see what directory you're in based on what is written next to your cursor.
Windows:
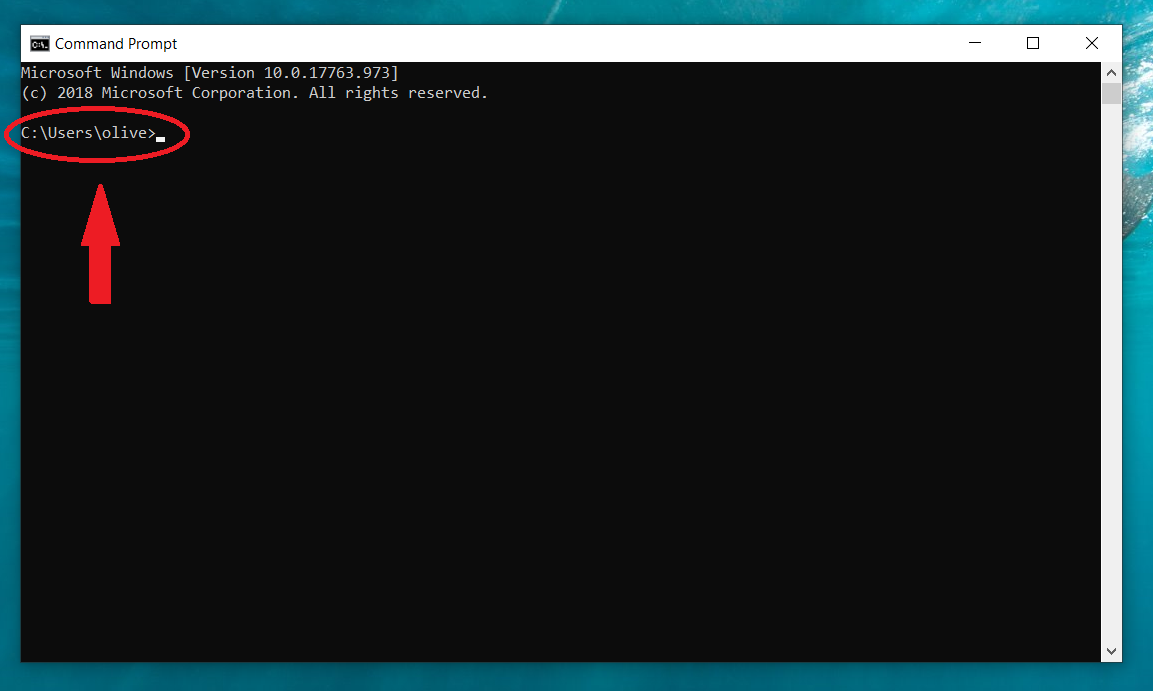
Mac: (Mac is a bit harder to see, it's inbetween the words)
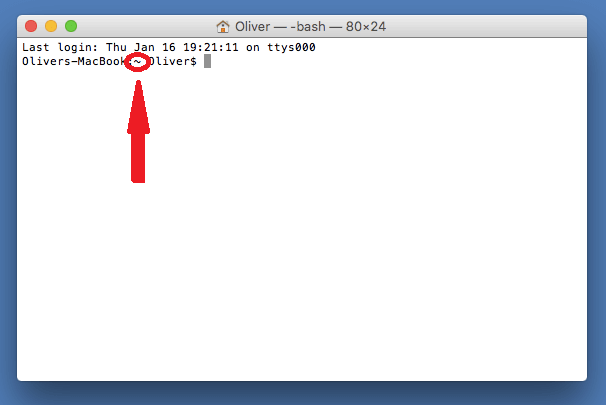
Now when we type in:
And press Enter, nothing will happen, that's because cd needs to know where you want to go. This is perfect for us to now say where we want to create the folder to store our code. I want to store it in Documents, so I'm going to type:
On Windows:
On Mac:
Then when we press Enter, and look at where we are at now, we'll see we've changed our location:
Windows:
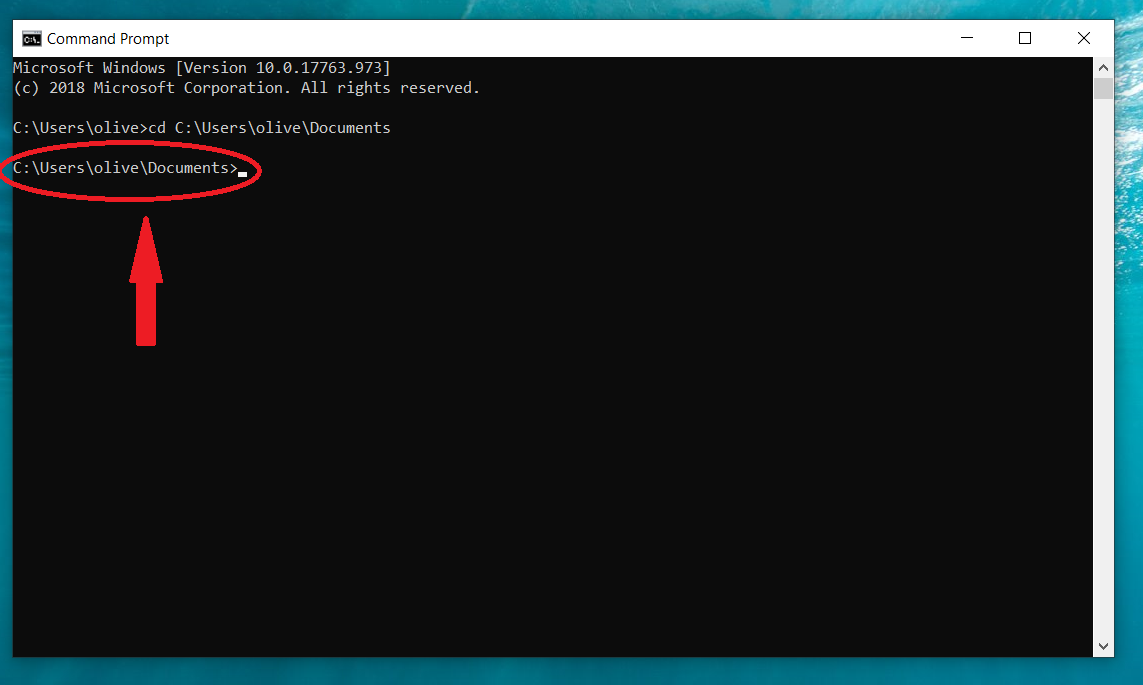
Mac:
3. Now that we can change to a directory of our choosing, we are ready to create the folder that will store how code. To do this we have to use a new command in the command line. It is:
This command makes a directory. Like cd we have to give it another bit of information. In this case it's the name we want to call it. I'm going to call it myCode. So if we type and press Enter:
We will create a folder where our command line was at. In my case it was in C:\Users\olive\Documents on Windows & ~/Documents on mac.
Windows:
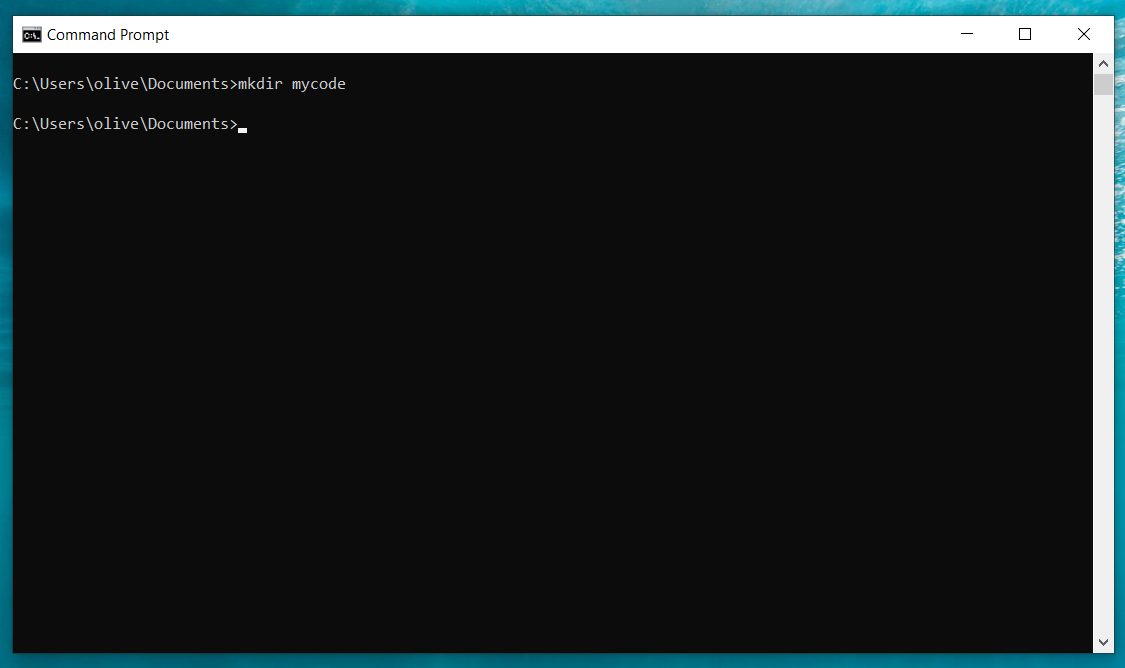
Mac:
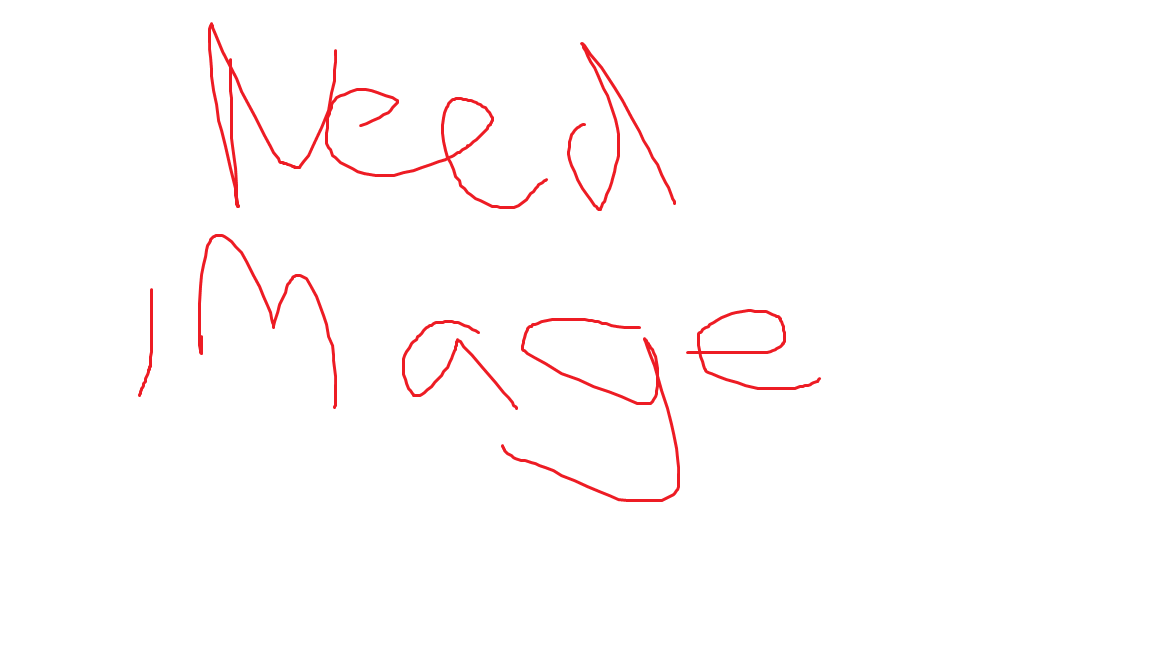
Now if we go have a look in Documents, we'll find our new folder mycode.
Windows:
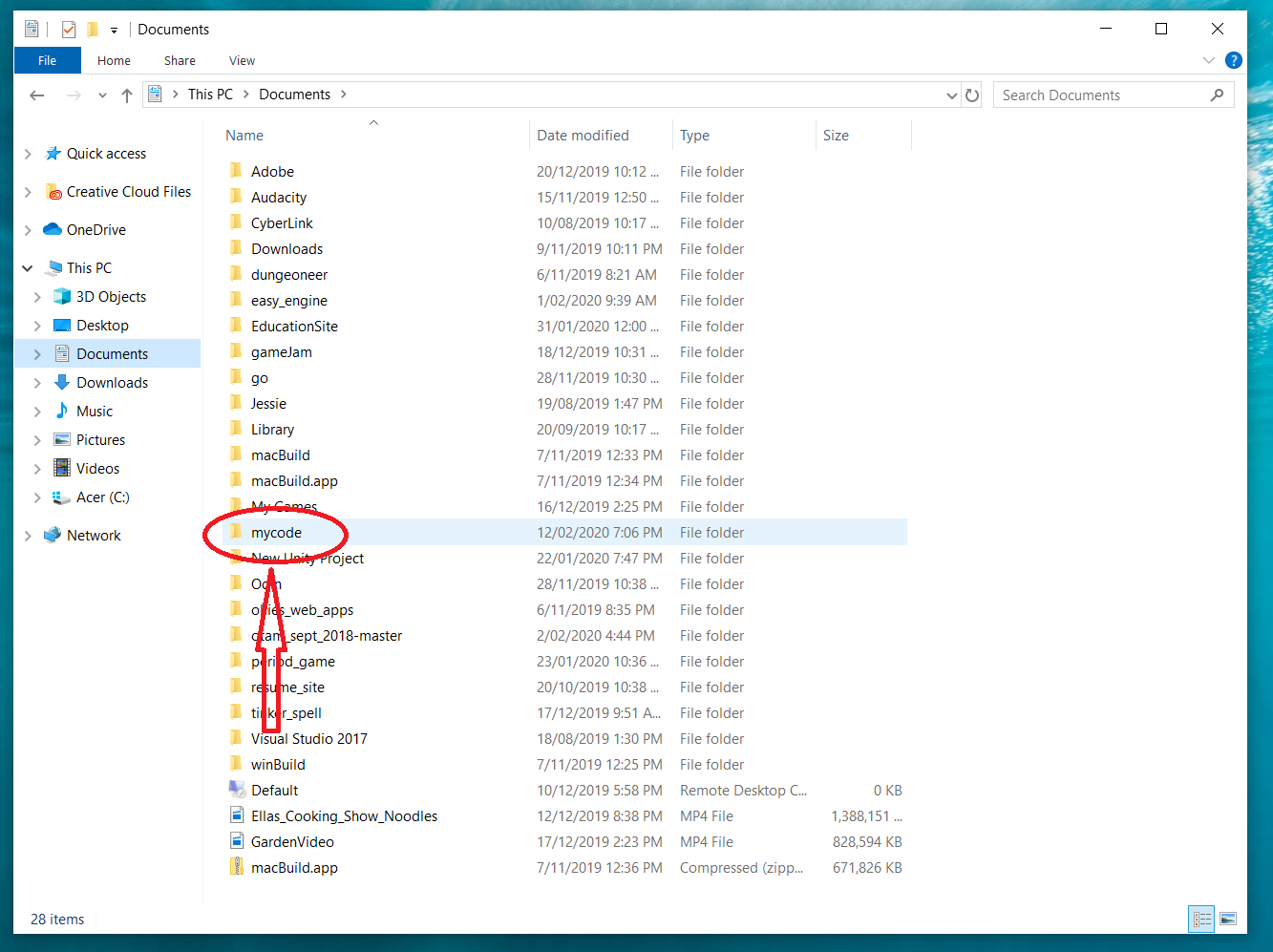
Mac:
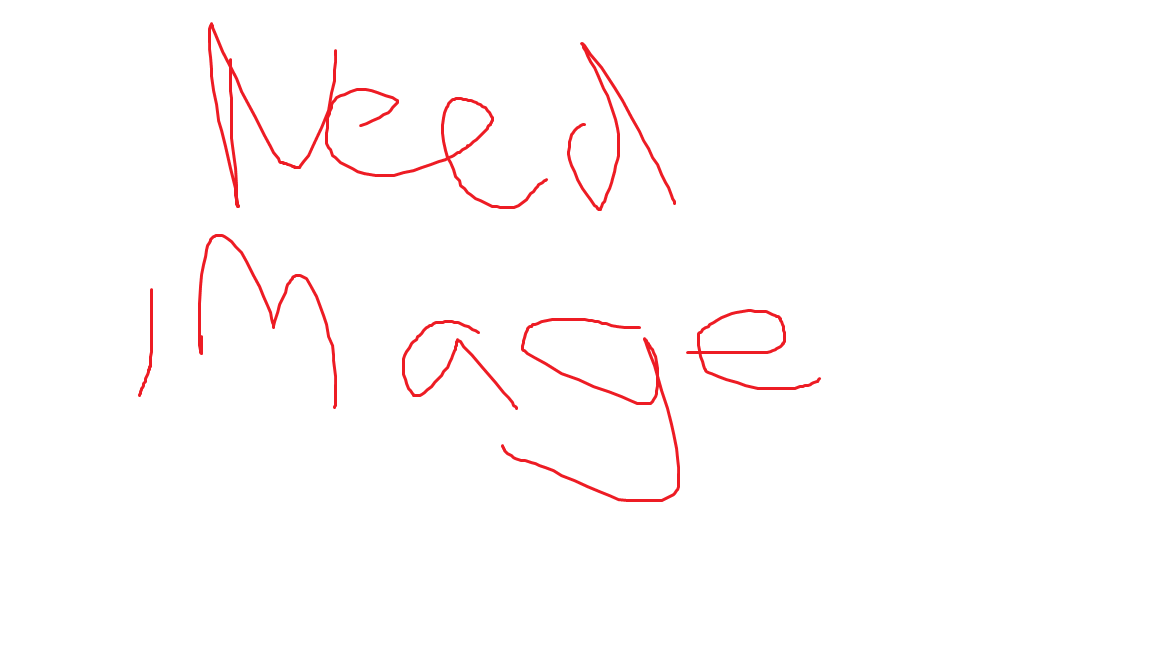
Ok, nearly there! Now we can use our cd command to change directory into our newly created folder.
You'll see we now moved into the mycode folder on the commmand line.
Windows:
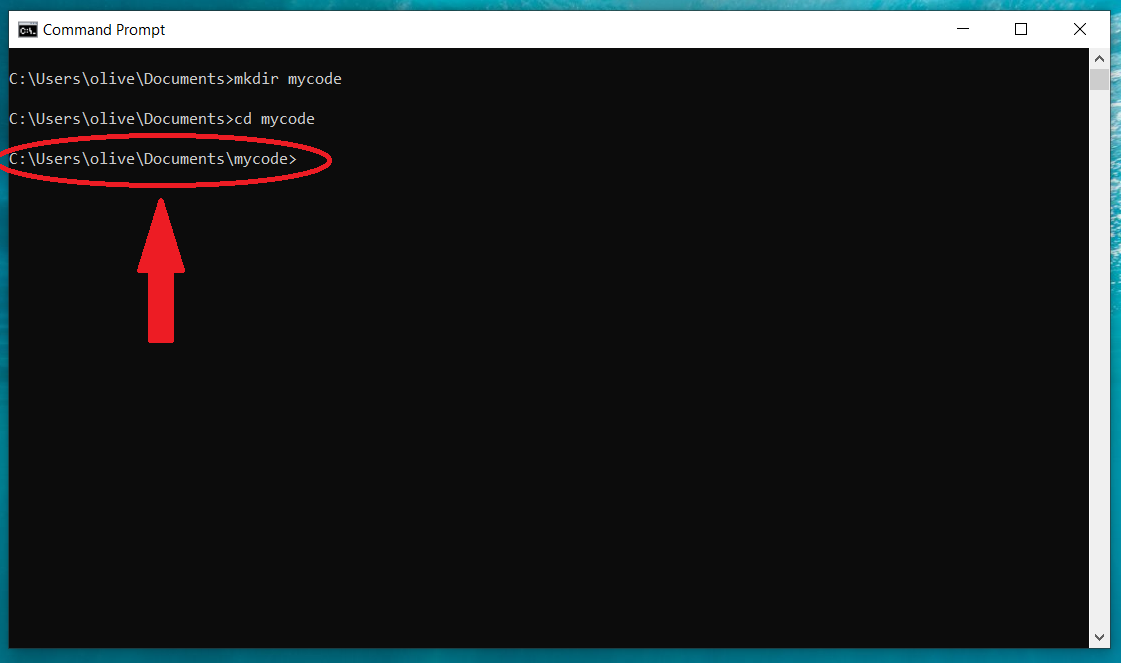
Mac:
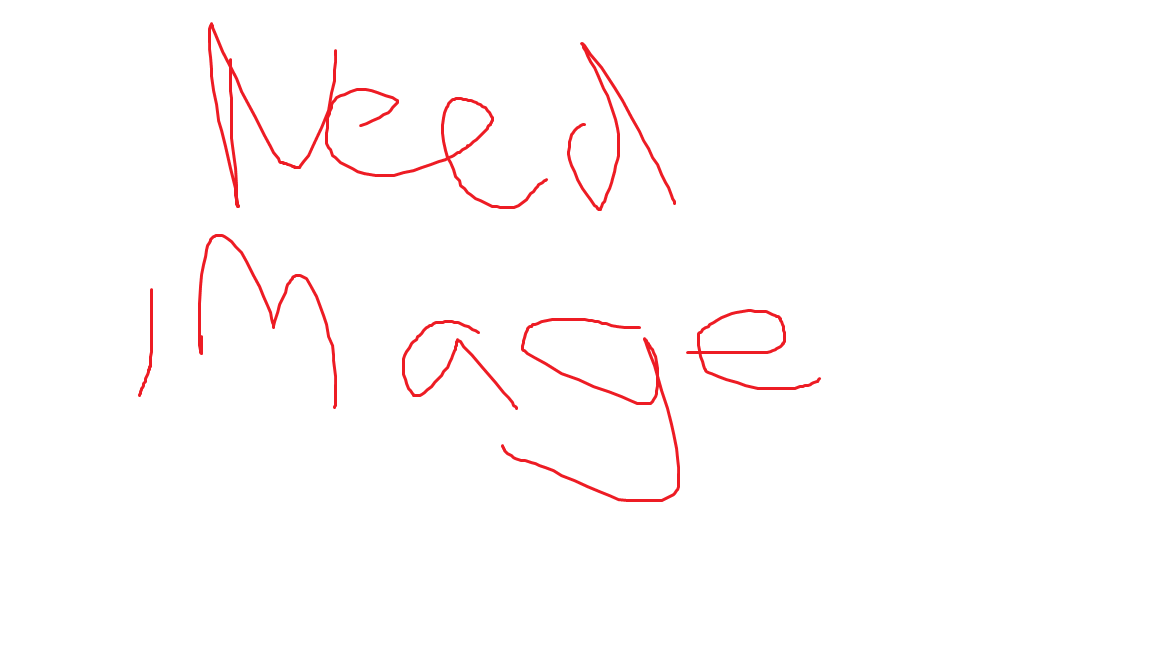
Yay! Now that we're in our folder we want our code to live in, we are ready to create our first .cpp file. That is, a file that we will right our c++ code in. We can do this from the command line, but we can accidently overwrite files that are already there. So it's much safer to do in the text editor we are using. To do this in Sublime text, just go File -> New File, and save it in the folder we've just created. Find out how to do this in the text editor your using.
And now if we have a look in the file explorer in the mycode folder, we'll see our first file is there.
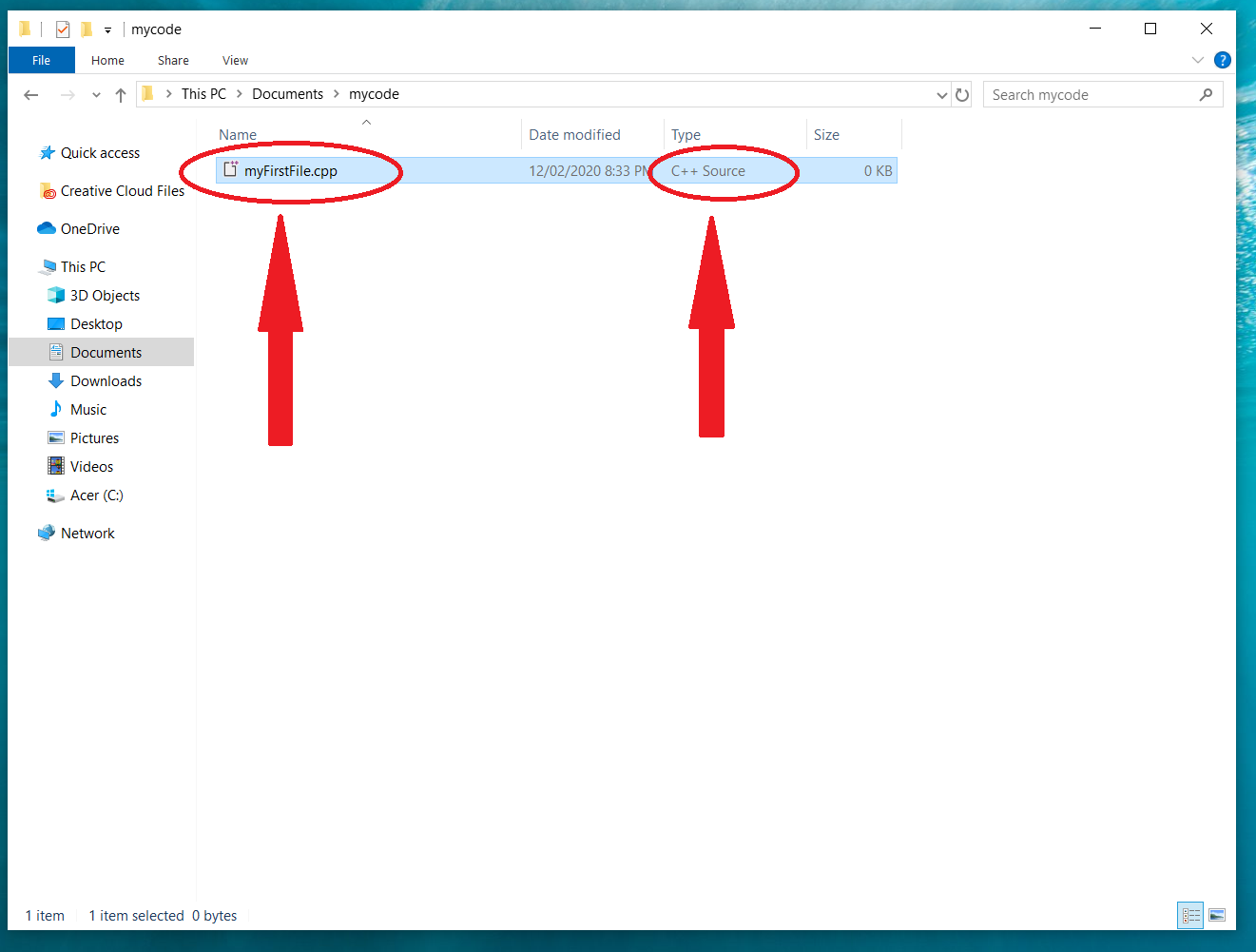
Awesome! We created our first .cpp file, learnt how to create folders from the command line using mkdir, that the command line is always located at some directory, & navigate around the computer on the command line using the cd command. Now that we've created our file, we can actually start writing some code into it. In the next lesson we'll add out entry point function in & then compile it, turning it into a real computer program.
Quick Quiz:
What file extension does a C++ file have? Click to see
cpp
What slash do file names on Windows use? What slash do file names on Mac use? Click to see
Windows uses back slashes \
Mac uses forward slashes /
What is the command to change the directory the command line is currently located at? Click to see
cd
What is the full command to go up one directory on the command line? Click to see
cd ..
What is the command to create a folder on the command line? Click to see
mkdir
What other bit of information does this command require to work? Click to see
The name of the folder you want to make. eg. mkdir myNewFolder