Lesson 3: Variables & Functions
In the last lesson we introduced the entry point function called the main function. This is where our program starts running. It is a bit complicated for a begining function, so in this lesson we'll go over some more examples of functions so you can get a better grasp on them.
As we learnt in the last lesson, functions have a name, input variables, only one or no ouput variables, and the actual code (or the body of the function, where the actual code goes). Here is an example of a function:
int leftOverCarrots = totalCarrots - numberOfCarrots;
return leftOverCarrots;
}
Here our function is doing something, in this case telling the rabbit to eat carrots. Functions are the verbs of our program, they do stuff for us. Variables on the other hand are the nouns of our program. They represent things. We give them to functions to do things on them.
In this example we're passing into the function the number of carrots we want the rabbit to eat. Int being the type of the variable - which is an Integer (a negative or positive whole number). On the left hand side of rabbitEatCarrots( we're also saying this function must return something for us. Meaning in our function body we must use the keyword return followed by an integer variable. In this case we're returning the total number of carrots.
Once we declare what's expected to come in (the input variables) and what's expected to come out (the output variables), we can actually write what this function is going to do, in this case eat some carrots. You can see we're creating a new variable called leftOverCarrots. And the value of this variable will be the sum that we calculate on the right - the total number of carrots minus the carrots we want to eat.
We're then returning this result back out of the function so it can be used by function that called this function or the outer scope. This could look like this:
int carrotCount = 10;
carrotCount = rabbitEatCarrots(2, carrotCount);
print(carrotCount);
}
Here is our outer function that has it's own function declaration - void main(void). This is saying the function is called main and it doesn't have any input or output variables, denoted by void. It's then creating a variable of type int (a number) called carrot count, and making it equal 10. This represents the starting number of carrots the rabbit has.
It then calls our rabbitEatCarrots function passing in the two input variables it needs - the number of carrots that are being eaten and the total number of carrots the rabbit has. When the program gets to this point, it will enter the rabbitEatCarrots function, calculate the number of carrots that are left, and return the value, assigning the new value to carrot count.
We can then see what this value is by calling another function print which would output our final value which would be 8.
You can see in this example that a program moves from top to bottom inside a function. Just like we read from left to right, top to down in the English language, a program will move from top to bottom in a function. If it reaches a function, our program will enter that, start from the top and go till the bottom, then will jump back to where it was on the function it was in orignially.
A program is built up of functions that operate on variables. When we run our program, it enters in at the entry point and moves from top to bottom of functions, entering and exiting other functions as they are called, until it gets to the end and exits. This is what all computer programs do, and games are built.
Let's write this now in a c program like we've learnt in previous lessons.
//code will go here
return 0;
}
We've got our entry point to the program like we've done before. Next we're going to add our code for the rabbit's carrots.
int carrotCount = 10;
carrotCount = rabbitEatCarrots(2, carrotCount);
printf("%d", carrotCount);
return 0;
}
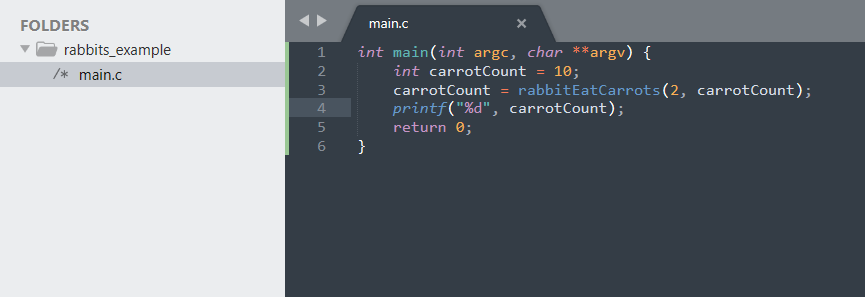
What the code would look like in our text editor.
Now if we compile this with our cl command we'll get an error.
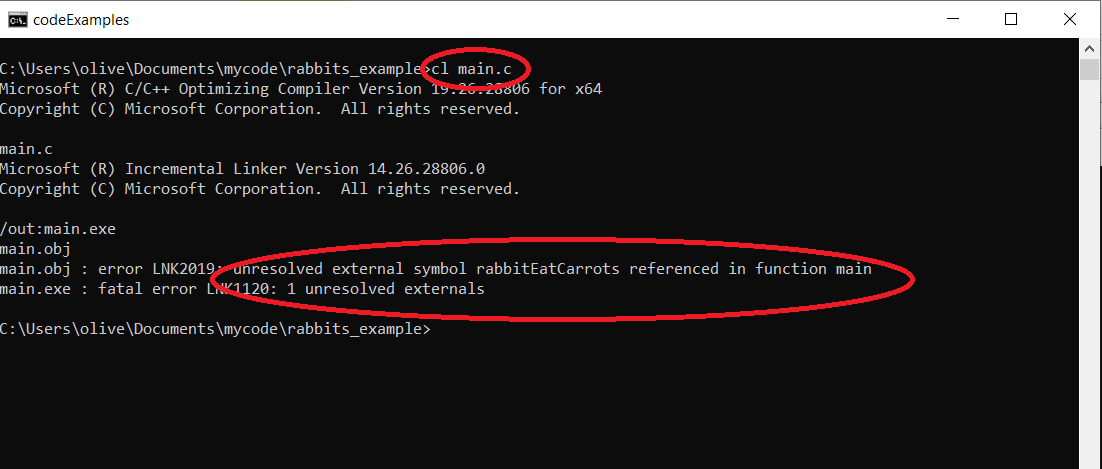
It says it can't find the function rabbitEatCarrots. Why would that be? It's because we haven't made it. We're saying use this function, the compiler then looks for it but can't find it. We have to write it.
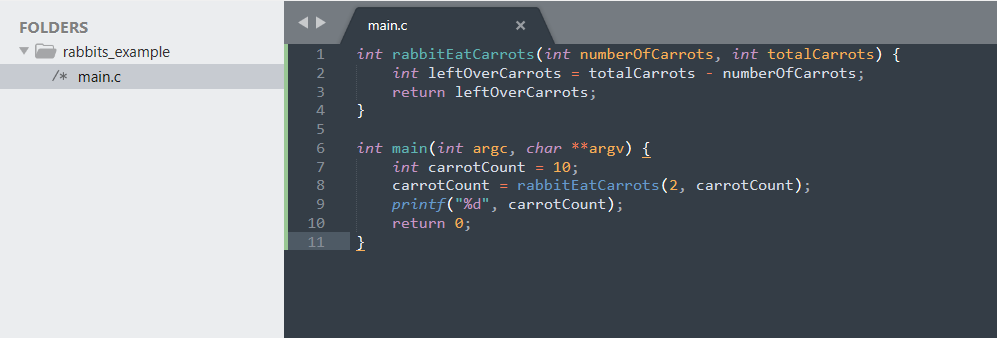
Once we add it above the main function, it should look like this.
We can then compile it using our cl command again - cl main.c and it should compile. We can then run our command by running the .exe the compiler created for us - main.exe
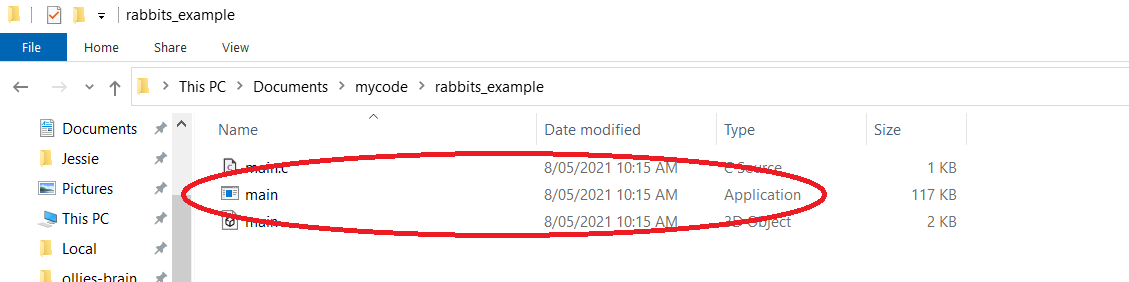
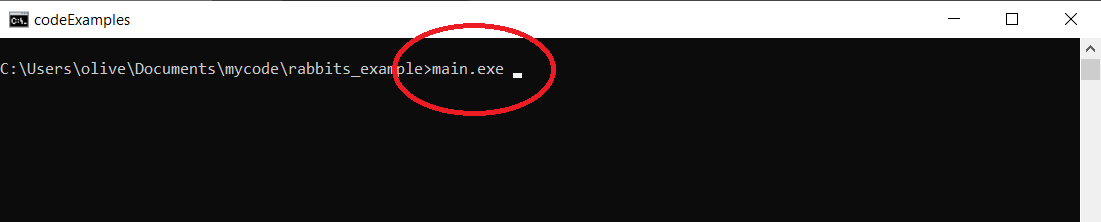
Running our program
One we press enter our program will run, executing the code we've written.
As you can see it output 8 - how many carrots were left after the rabbit ate 2.
Awesome! We created a program that ran real code and had more than one function in it. We're one our way to making a gam engine!